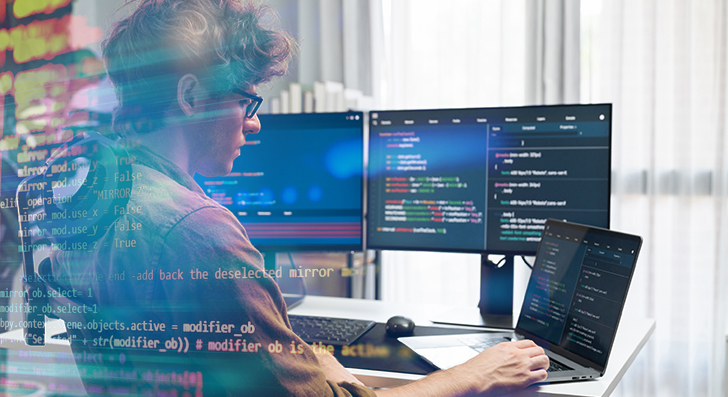
Scalability implies your software can take care of progress—much more users, extra knowledge, and a lot more site visitors—with out breaking. To be a developer, constructing with scalability in mind will save time and strain later on. Here’s a transparent and sensible guideline that may help you commence by Gustavo Woltmann.
Design for Scalability from the beginning
Scalability isn't something you bolt on later on—it should be aspect of one's system from the beginning. Quite a few applications fall short whenever they grow rapid simply because the initial design can’t take care of the additional load. Like a developer, you might want to Feel early about how your technique will behave stressed.
Begin by coming up with your architecture to become versatile. Stay clear of monolithic codebases the place everything is tightly connected. Alternatively, use modular structure or microservices. These patterns split your application into smaller, impartial sections. Each module or provider can scale on its own with no influencing the whole program.
Also, contemplate your databases from day 1. Will it will need to deal with 1,000,000 buyers or simply a hundred? Select the ideal type—relational or NoSQL—depending on how your knowledge will grow. System for sharding, indexing, and backups early, even if you don’t need to have them still.
A further important stage is in order to avoid hardcoding assumptions. Don’t generate code that only is effective under present-day disorders. Think about what would occur In case your user base doubled tomorrow. Would your app crash? Would the databases decelerate?
Use style and design patterns that assistance scaling, like message queues or event-pushed methods. These assist your app handle more requests without acquiring overloaded.
Once you Construct with scalability in mind, you are not just making ready for achievement—you happen to be lowering potential headaches. A well-planned system is less complicated to keep up, adapt, and expand. It’s much better to prepare early than to rebuild afterwards.
Use the best Database
Choosing the correct databases is often a essential Portion of building scalable purposes. Not all databases are created the identical, and using the Erroneous one can slow you down or even bring about failures as your app grows.
Start by knowledge your info. Is it remarkably structured, like rows within a desk? If Indeed, a relational database like PostgreSQL or MySQL is a good in shape. They're strong with associations, transactions, and consistency. Additionally they support scaling approaches like study replicas, indexing, and partitioning to take care of additional visitors and details.
Should your details is much more adaptable—like user action logs, products catalogs, or paperwork—take into consideration a NoSQL possibility like MongoDB, Cassandra, or DynamoDB. NoSQL databases are far better at managing big volumes of unstructured or semi-structured knowledge and will scale horizontally a lot more conveniently.
Also, think about your examine and write designs. Are you currently undertaking many reads with fewer writes? Use caching and browse replicas. Are you presently handling a large produce load? Look into databases that will cope with large produce throughput, or simply event-based mostly facts storage units like Apache Kafka (for temporary info streams).
It’s also sensible to Assume in advance. You might not want State-of-the-art scaling options now, but choosing a database that supports them indicates you won’t want to change later on.
Use indexing to speed up queries. Keep away from unwanted joins. Normalize or denormalize your information according to your accessibility patterns. And often keep an eye on database functionality while you increase.
In a nutshell, the best databases relies on your application’s composition, pace demands, And just how you assume it to increase. Get time to choose wisely—it’ll save a lot of problems later.
Optimize Code and Queries
Fast code is essential to scalability. As your application grows, every single tiny delay provides up. Improperly prepared code or unoptimized queries can slow down overall performance and overload your program. That’s why it’s vital that you Develop efficient logic from the beginning.
Start off by creating clean, simple code. Stay clear of repeating logic and take away anything at all unnecessary. Don’t choose the most complex Option if an easy one is effective. Maintain your functions shorter, targeted, and easy to check. Use profiling resources to uncover bottlenecks—sites the place your code will take too very long to run or takes advantage of excessive memory.
Next, check out your database queries. These generally slow points down in excess of the code itself. Ensure that Every question only asks for the data you really need. Keep away from SELECT *, which fetches almost everything, and instead decide on specific fields. Use indexes to hurry up lookups. And steer clear of undertaking a lot of joins, Specifically throughout large tables.
In case you notice the identical facts becoming requested time and again, use caching. Store the effects temporarily making use of instruments like Redis or Memcached so you don’t must repeat high-priced functions.
Also, batch your databases operations once you can. As an alternative to updating a row one after the other, update them in teams. This cuts down on overhead and makes your application additional efficient.
Remember to check with huge datasets. Code and queries that get the job done great with 100 records may crash after they have to manage one million.
Briefly, scalable apps are quick apps. Keep your code tight, your queries lean, and use caching when necessary. These measures support your software keep clean and responsive, whilst the load will increase.
Leverage Load Balancing and Caching
As your application grows, it's to take care of extra buyers and more traffic. If everything goes through one server, it'll rapidly become a bottleneck. That’s where by load balancing and caching are available. Both of these instruments support keep the application rapidly, steady, and scalable.
Load balancing spreads incoming site visitors across multiple servers. In lieu of a person server accomplishing the many operate, the load balancer routes consumers to various servers based on availability. This suggests no one server receives overloaded. If a single server goes down, the load balancer can deliver visitors to the Some others. Equipment like Nginx, HAProxy, or cloud-primarily based solutions from AWS and Google Cloud make this straightforward to create.
Caching is about storing information quickly so it could be reused rapidly. When buyers ask for the exact same details again—like an item webpage or a profile—you don’t should fetch it from your databases each and every time. You can provide it in the cache.
There's two frequent different types of caching:
1. Server-side caching (like Redis or Memcached) outlets info in memory for speedy accessibility.
two. Consumer-facet caching (like browser caching or CDN caching) shops static documents close to the consumer.
Caching reduces database load, increases speed, and would make your app extra effective.
Use caching for things which don’t alter generally. And always be sure your cache is current when info does transform.
In brief, load balancing and caching are uncomplicated but powerful equipment. Alongside one another, they help your app handle a lot more people, stay quickly, and Get well from problems. If you plan to increase, you would like each.
Use Cloud and Container Equipment
To develop scalable purposes, you website need resources that allow your application improve easily. That’s exactly where cloud platforms and containers are available in. They provide you overall flexibility, cut down set up time, and make scaling A lot smoother.
Cloud platforms like Amazon Net Companies (AWS), Google Cloud System (GCP), and Microsoft Azure Enable you to hire servers and products and services as you need them. You don’t have to purchase hardware or guess long term ability. When website traffic improves, you could increase extra means with just some clicks or quickly using vehicle-scaling. When traffic drops, you can scale down to economize.
These platforms also offer services like managed databases, storage, load balancing, and stability instruments. You may focus on building your application in place of taking care of infrastructure.
Containers are One more crucial Instrument. A container packages your application and almost everything it has to run—code, libraries, configurations—into just one unit. This makes it quick to maneuver your app between environments, from your notebook on the cloud, without having surprises. Docker is the most popular Software for this.
Whenever your app takes advantage of a number of containers, resources like Kubernetes help you regulate them. Kubernetes handles deployment, scaling, and recovery. If one aspect of the application crashes, it restarts it routinely.
Containers also allow it to be straightforward to different areas of your application into companies. You are able to update or scale pieces independently, which can be perfect for functionality and reliability.
In a nutshell, using cloud and container instruments indicates you may scale quick, deploy quickly, and Recuperate promptly when issues transpire. If you would like your application to expand without the need of limitations, start out utilizing these instruments early. They save time, lessen risk, and enable you to continue to be focused on creating, not correcting.
Monitor Every little thing
When you don’t keep track of your application, you gained’t know when matters go Incorrect. Monitoring helps you see how your app is undertaking, location problems early, and make greater selections as your application grows. It’s a key Portion of constructing scalable units.
Start by tracking simple metrics like CPU utilization, memory, disk Place, and reaction time. These show you how your servers and services are performing. Equipment like Prometheus, Grafana, Datadog, or New Relic can assist you collect and visualize this information.
Don’t just keep track of your servers—keep an eye on your application way too. Control how much time it's going to take for users to load pages, how often mistakes take place, and in which they arise. Logging instruments like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s occurring within your code.
Put in place alerts for critical challenges. One example is, If the reaction time goes higher than a Restrict or maybe a provider goes down, you must get notified quickly. This will help you correct concerns quickly, frequently before users even detect.
Checking is additionally helpful whenever you make changes. When you deploy a whole new characteristic and see a spike in faults or slowdowns, you may roll it back again prior to it leads to real destruction.
As your app grows, visitors and details enhance. Without having checking, you’ll overlook signs of issues until finally it’s as well late. But with the ideal equipment in place, you keep in control.
Briefly, monitoring can help you keep your application dependable and scalable. It’s not pretty much spotting failures—it’s about being familiar with your program and ensuring that it really works effectively, even stressed.
Last Views
Scalability isn’t just for significant organizations. Even compact apps will need a strong foundation. By building very carefully, optimizing sensibly, and using the appropriate tools, it is possible to Establish apps that increase smoothly without having breaking stressed. Start tiny, Imagine large, and Create good.